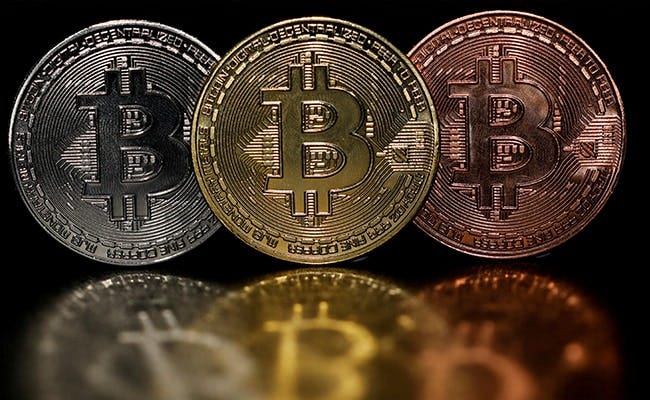
What is Cryptocurrency?
A cryptocurrency is a digital or virtual currency that is secured by cryptography, which makes it nearly impossible to counterfeit or double-spend. Many cryptocurrencies are decentralized networks based on blockchain technology — a distributed ledger enforced by a disparate network of computers. A defining feature of cryptocurrencies is that they are generally not issued by any central authority, rendering them theoretically immune to government interference or manipulation.
After a brief introduction of cryptocurrency, the question that arises is, what we will do in this article?
Before Answering the above question, detailed video series of this project is on youtube
Now let’s start
We gonna create a personal portfolio project to manage and track the prices of all the cryptocurrencies you own. This is a long project so covering all the things in one article is not possible so make sure to follow the page so that you do not miss the future article.
In this article, we will simply create a project where we will fetch the top 100 currency according to the market cap and render them in the django template.
Requirements for this project
- Django must be installed.
- Celery
- Redis
- Django-channels
- Coingecko API
Setup a basic Django project and do the necessary configuration and setting for the Django templates and apps. To follow the project from scratch follow me on youtube.
Now open the views.py file and start typing the code for fetching the data from the API.
from django.shortcuts import render
import requests
from django.http import HttpResponse
# Create your views here.
def indexView(request):
data_url = 'https://api.coingecko.com/api/v3/coins/markets? vs_currency=usd&order=market_cap_desc&per_page=100&page=1&sparkline=false'
raw_data = requests.get(data_url).json()
return render(request, 'crypto/index.html', context={'datas': raw_data})
Now it’s time for URL mapping.
### urls.pyfrom crypto.views import indexViewurlpatterns = [..... path('', indexView, name='home'),.....]
Now open your index.html file and access the data we are sending from the views.
### index.html{% block content %}
<div class="jumbotron">
<h4>GET THE CRYPTO DATA YOU NEED</h4>
</div>
<div class="container">
<table class="table table-striped table-dark">
<thead>
<tr>
<th scope="col">Logo</th>
<th scope="col">Name</th>
<th scope="col">Rank</th>
<th scope="col">Market cap</th>
<th scope="col">Price</th>
</tr>
</thead>
<tbody>
{% if datas %}
{% for data in datas %}
<tr>
<td><img src="{{data.image}}" height="70px" alt="Coin Logo"></td>
<td class="align-middle">{{data.name}}</td> <td class="align-middle">{{data.market_cap_rank}}</td>
<td class="align-middle">
{{data.market_cap}}</td>
<td class="align-middle">{{data.current_price}} USD</td>
</tr>
{% endfor %}
{% else %}
<p>Nothing Here to show</p>
{% endif %}
</tbody>
</table>
</div>
{% endblock %}
That’s it, refresh the page and you can see all the 100 cryptocurrencies in decreasing order according to market cap.
So thank you for reading this article and please follow on youtube and medium to stay updated and get notified for the next part of this tutorial.
Cheers!!!
Happy coding.